About Delegates
You can think of a delegate as an object that holds one or more methods. Normally, of course, you wouldn’t think of "executing" an object,
but a delegate is different from a typical object. You can execute a delegate, and when you do so, it executes the method or methods that it "holds".
A delegate is a user-defined type, just as a class is a user-defined type. But whereas a class represents a collection of data and methods, a delegate holds one or more methods and a set of predefined operations.
You use a delegate by performing the following steps.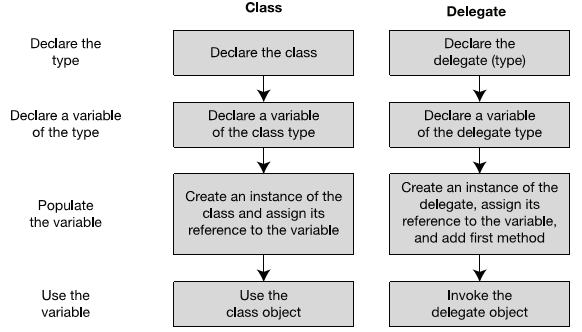
In the other words, you can think a delegate as an object that contains an ordered list of methods with the same signature and return type.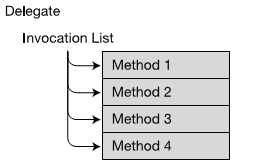
A delegate is a user-defined type, just as a class is a user-defined type. But whereas a class represents a collection of data and methods, a delegate holds one or more methods and a set of predefined operations.
You use a delegate by performing the following steps.
- Declare a delegate type. A delegate declaration looks like a method declaration, except that it doesn’t have an implementation block.
- Declare a delegate variable of the delegate type.
- Create an object of the delegate type and assign it to the delegate variable. The new delegate object includes a reference to a method that must have the same signature and return type as the delegate type defined in the first step.
- You can optionally add additional methods into the delegate object. These methods must have the same signature and return type as the delegate type defined in the first step.
- Throughout your code you can then invoke the delegate, just as it if it were a method. When you invoke the delegate, each of the methods it contains is executed.
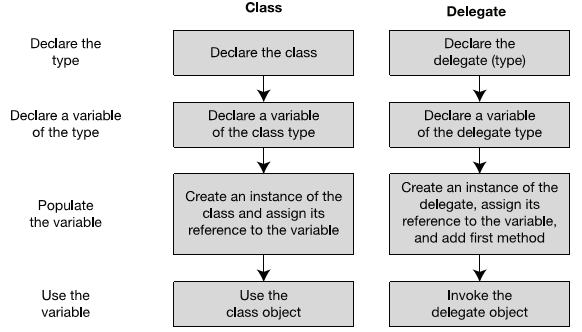
In the other words, you can think a delegate as an object that contains an ordered list of methods with the same signature and return type.
- The list of methods is called the invocation list.
-
Methods held by a delegate can be from any class or struct, instance methods or static methods, as long as they match both return type and parameter
list include
ref
orout
modifier. - When a delegate is invoked, each method in its invocation list is executed.
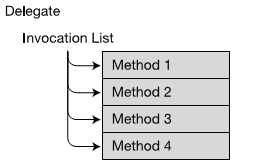
Declaring, Instantiating and Accessing Delegate
Declaring Delegate
The declaration of a delegate type looks much like the declaration of a method, in that it has both a return type and a signature. The return type and signature specify the form of the methods that the delegate will accept.delegate [Return Type] [Delegate Name]( [Parameter List] );
Creating and Assigning Delegate Object
A delegate is a reference type and therefore has both a reference and an object. After a delegate type is declared, you can declare variables and create objects of the type. See Following sytax:[Delegate Name] [Instance Name];
[Delegate Name] [Instance Name] = new [Delegate Name]([Function Name]);
[Delegate Name] [Instance Name] = [Function Name];
Putting All Together
delegate int operation(int x,int y); //declaring delegate static class mathOperator { public static int add(int x,int y) { return x + y; } public static int multiply(int x, int y) { return x * y; } } class Program { static void Main(string[] args) { int x = 5; int y = 7; operation myAddOperator = new operation(mathOperator.add); //instantiating delegate operation myMultiplyOperator = mathOperator.multiply; //instantiating delegate Console.WriteLine("myAddOperator(" + x + "," + y + ") = " + myAddOperator(x, y)); //invoking delegate Console.WriteLine("myMultiplyOperator(" + x + "," + y + ") = " + myMultiplyOperator(x, y)); //invoking delegate } }
The code will display following output:
myAddOperator(5,7) = 12
myMultiplyOperator(5,7) = 35
myMultiplyOperator(5,7) = 35
Adding and Removing Methods to Delegates
Adding Methods
Although you saw in the previous section that delegates are, in reality, immutable, C# provides syntax for making it appear that you can add a method to a delegate, using the+=
operator.
For example:
operation myOperator = mathOperator.add; myOperator += mathOperator.multiply;

Removing Methods
You can also remove a method from a delegate, using the-=
operator. For example:
myOperator -= mathOperator.multiply;
There are some things to remember when removing methods:
-
If there are multiple entries for a method in the invocation list, the
-=
operator starts searching at the bottom of the list and removes the first instance of the matching method it finds. - Attempting to delete a method that is not in the invocation list has no effect.
-
Attempting to invoke an empty delegate throws an exception. You can check whether a delegate’s invocation list is empty by comparing the delegate
to
null
. If the invocation list is empty, the delegate isnull
.
delegate void operation(int x,int y); static class mathOperator { public static void add(int x,int y) { Console.WriteLine(x + " + " + y + " = " + (x + y)); } public static void multiply(int x, int y) { Console.WriteLine(x + " * " + y + " = " + (x * y)); } } class Program { static void Main(string[] args) { int x = 5; int y = 7; operation myOperator = new operation(mathOperator.add); Console.WriteLine("Adding multiply operation"); myOperator += mathOperator.multiply; myOperator(x, y); Console.WriteLine("Removing multiply operation"); myOperator -= mathOperator.multiply; myOperator(x, y); Console.Read(); } }
The code will display following output:
Adding multiply operation
5 + 7 = 12
5 * 7 = 35
Removing multiply operation
5 + 7 = 12
5 + 7 = 12
5 * 7 = 35
Removing multiply operation
5 + 7 = 12
0 comments:
Post a Comment